Assembly Language Program To Read A String And Display
Assembly language comment begins with a semicolon (;). It may contain any printable character including blank. It can appear on a line by itself, like −; This program displays a message on screen or, on the same line along with an instruction, like −. Add eax, ebx; adds ebx to eax Assembly Language Statements. We specify the character to be displayed. This is done by storing the character’s ASCII code in a specific 8086 register. In this case we use the dl register, i.e. We use dl to pass a parameter to the output subprogram.
- Assembly Language Program To Read A String And Display Key
- Assembly Language Program To Read A String And Display The Image
Write an Assembly Language Program to Display a String
Object:
Write an Assembly Language Program to Display a String / Text / Hello World.
Code:
.MODEL SMALL
.STACK 64
.DATA
MSG DB ‘Welcome to codeboks.com’,’$’
.CODE
MAIN PROC FAR
MOV AX,@DATA
MOV DS,AX
CALL D
MOV AH,4CH
INT 21H
MAIN ENDP
D PROC
MOV AH,09
MOV DX,OFFSET MSG
INT 21H
RET
D ENDP
END MAIN
2 4 6 8 10 12 14 16 18 20 22 24 | .STACK64 MSG DB'Welcome to codeboks.com','$' .CODE MAIN PROC FAR MOV AX,@DATA CALLD INT21H MAIN ENDP DPROC MOV DX,OFFSET MSG RET END MAIN |
Command Prompt:
DOSBOX:
Character Output
mov dl, ‘a‘ ; dl = ‘a‘
mov ah, 2h ; character output subprogram
int 21h ; call ms-dos output character
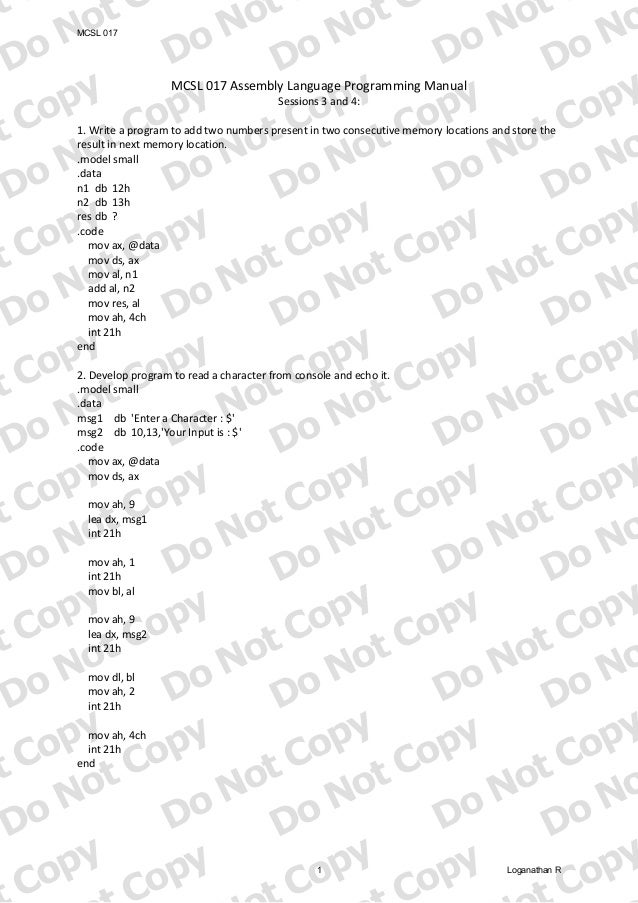
mov ah, 1h ; keyboard input subprogram
; character is stored in al
mov c, al ; copy character from al to c
Example 3: Reading and displaying a character:
mov ah, 1h ; keyboard input subprogram
mov dl, al ; copy character to dl
mov ah, 2h ; character output subprogram
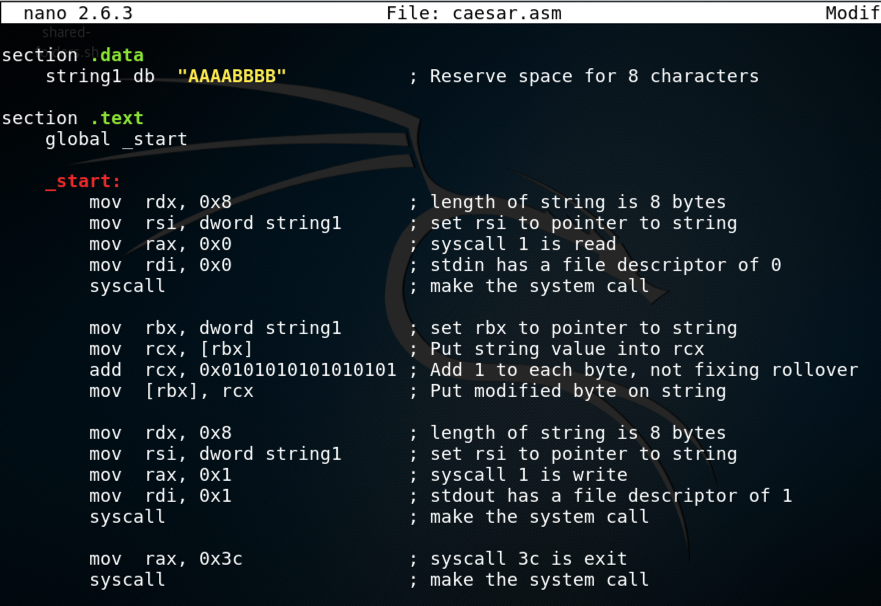
int 21h ; display character in dl
String output
1.Under the data segment, we define a variable to serve as the starting address for the string, array of characters or ASCII codes ending with '$'.
e.g message DB 'Your text goes here','$'
OR message DB 'Y','o','u','r',' ','t','e','x','t',' ','g','o','e','s',' ','h','e','r','e','$' ;Pretty long huh?
OR message DB 89,111,117,114, ... ,$ ;In ASCII codes.
where
message is the name of the variable,
DB means 'Define byte',
'$' indicates end of string, and
the digits are the ASCII code of the characters.
2. Under the code segment(also explained in next post), enter
MOV AX, @data
MOV DS, AX
to initialize the DS register
This moves the address of your data in memory to register DS.
then, offset the variable to DX for display
i.e MOV DX, OFFSET message
Example 4
.data

.code
start: MOV AX, @data
MOV DS, AX
MOV DX, offset notice ;Copies notice address to DX for display
MOV AH, 09H
INT 21H ;Calls MS-DOS to display string
MOV AH, 4C00H ;Returns control to MS-DOS
INT 21H
END start
String input
1.Under the data segment, we define the variable to contain the string.
e.g message DB ?
where
message is the name of the variable,
DB means 'Define byte',
? indicates the memory created should be empty.
2. Under the code segment(also explained in next post), enter
MOV AX, @data
MOV DS, AX
to initialize the DS register
This moves the address of your data in memory to register DS.
then, offset the variable to DX
i.e MOV DX, OFFSET message
Example 4
.data
value DB ?
.code
start: MOV AX, @data
MOV DS, AX
MOV DX, OFFSET value ;Copies notice address to DX for display
Assembly Language Program To Read A String And Display Key
MOV AH, 3FHINT 21H ;Calls MS-DOS to input string
MOV AH, 4C00H ;Returns control to MS-DOS
INT 21H
END start ;End of program.
;The program will simply prompt you to enter a string and exits.
Assembly Language Program To Read A String And Display The Image

;The string will be stored in memory starting from the address of the variable you declared(value).
;Note: in this case, CARRIAGE-RETURN (ASCII code 13) or LINE-FEED (ASCII code 10) indicates the end of the string.
2. 'Character input' retrieved from http://www.csi.ucd.ie